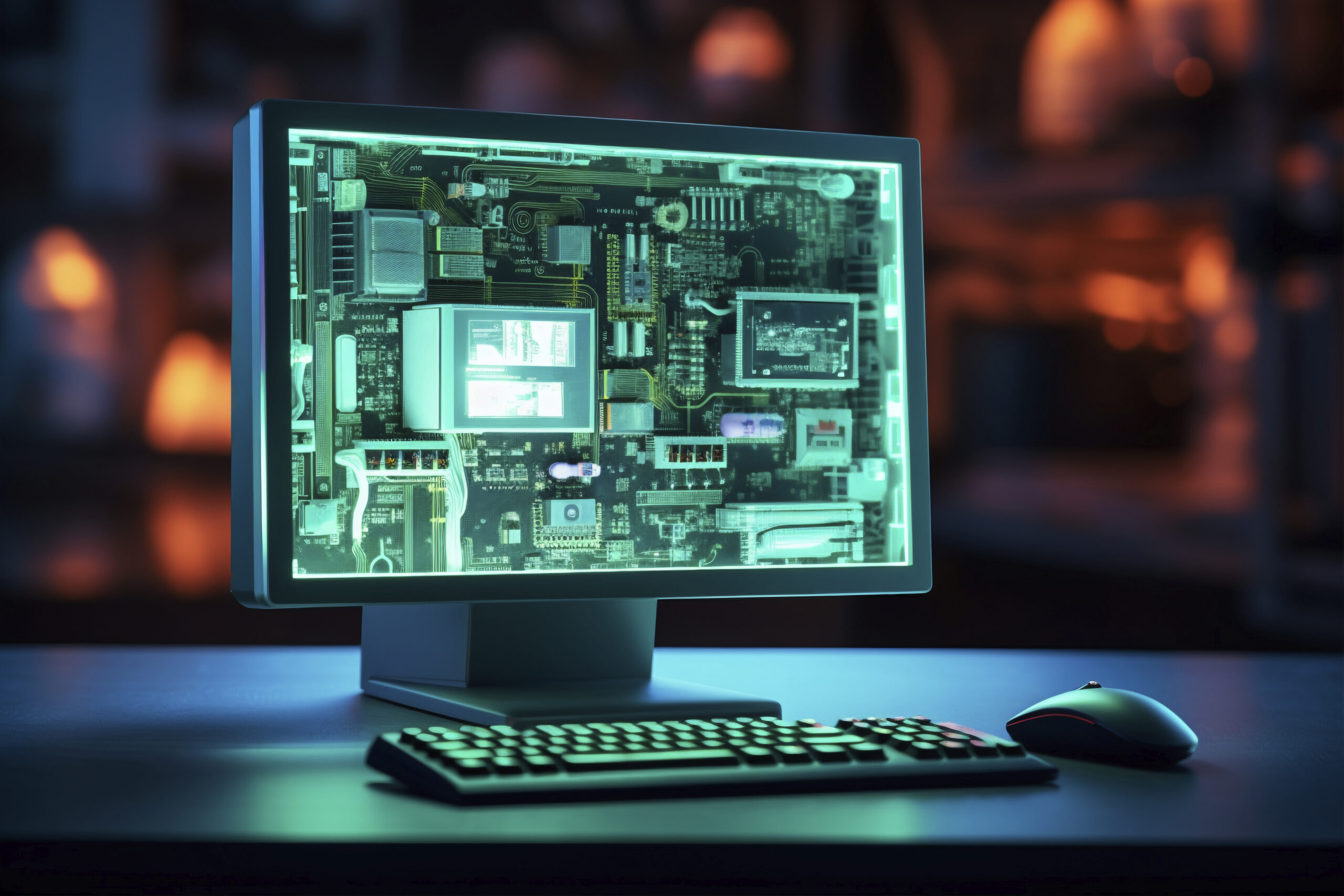
In the world of hardware design, SystemVerilog is a go-to language for engineers. It combines hardware description and verification capabilities, enabling efficient and accurate designs. Among its many features, tasks and functions stand out for their ability to modularize and simplify complex code. Let’s explore how these constructs streamline hardware design while adhering to the best practices for creating efficient and reusable code.
Why Modularization Matters in Hardware Design
Designing hardware involves managing intricate logic and numerous connections. Without modularization, debugging and maintaining such designs would be a nightmare. Modularization ensures:
- Code Reusability: Common logic can be reused across multiple modules.
- Ease of Debugging: Smaller, well-defined blocks are easier to troubleshoot.
- Improved Readability: Breaking down complex designs into simple units improves code clarity.
SystemVerilog’s tasks and functions are key tools for achieving these benefits.
What Are Tasks and Functions in SystemVerilog?
Tasks
A task is a reusable code block that performs operations. Tasks are capable of handling:
- Multiple inputs and outputs
- Timing control using
#
or@
- Complex logic that spans multiple cycles
Functions
A function is similar to a task but is more restricted. Functions:
- Always execute in a single simulation time unit
- Return a single value
- Cannot contain timing control
Key Differences Between Tasks and Functions
Feature | Tasks | Functions |
---|---|---|
Timing Control | Allowed | Not allowed |
Return Type | Can return values via output ports | Returns a single value directly |
Execution Time | Can span multiple simulation cycles | Must execute within one time unit |
Usage Complexity | Suitable for complex operations | Best for simple, combinational logic |
How Tasks and Functions Simplify Hardware Design
- Encapsulation of Repeated Logic
Tasks and functions encapsulate repetitive operations, reducing code duplication. For example, instead of writing the same set of operations in multiple places, you can create a task or function and call it whenever needed. Example:function [3:0] calculate_parity(input [3:0] data); calculate_parity = ^data; // XOR all bits endfunction
This function calculates the parity bit for a 4-bit input, simplifying the design wherever parity is required. - Improved Debugging and Maintenance
Isolating specific operations into tasks or functions makes debugging easier. Instead of tracing through hundreds of lines of code, you can focus on the specific task or function that performs the operation. - Enhanced Code Readability
Modularizing code improves readability. For example, instead of embedding complex logic in a module, you can use tasks and functions to make the design self-explanatory. - Reusable Building Blocks
Tasks and functions act as reusable blocks, saving time in large designs. For instance, a function that computes checksum can be used across multiple modules requiring error-checking capabilities.
Best Practices for Using Tasks and Functions
- Choose the Right Construct
- Use tasks for operations involving multiple outputs or timing control.
- Use functions for simple, combinational logic that returns a single value.
- Keep Tasks and Functions Short
Keep your tasks and functions focused on a single operation. This enhances maintainability and readability. - Name Them Clearly
Use descriptive names for tasks and functions to convey their purpose. For example, usecompute_crc
instead oftask1
. - Comment Extensively
Document the purpose, input, output, and constraints of tasks and functions to help other developers understand and use them correctly.
Example: Using Tasks and Functions Together
module arithmetic_operations;
// Task to calculate addition and subtraction
task automatic calculate(input [7:0] a, b, output [7:0] sum, diff);
sum = a + b;
diff = a - b;
endtask
// Function to multiply two numbers
function [15:0] multiply(input [7:0] a, b);
multiply = a * b;
endfunction
// Test the task and function
initial begin
reg [7:0] a = 8'd15, b = 8'd10;
reg [7:0] sum, diff;
reg [15:0] product;
// Call task
calculate(a, b, sum, diff);
$display("Sum: %d, Diff: %d", sum, diff);
// Call function
product = multiply(a, b);
$display("Product: %d", product);
end
endmodule
Output:
Sum: 25, Diff: 5
Product: 150
SEO Tips for Effective Ranking
To ensure this blog ranks well on search engines:
- Focus Keyword: Use “SystemVerilog tasks and functions” throughout the blog, maintaining a keyword density of 0.5-2.5%.
- Title Optimization: The title includes the primary keyword: SystemVerilog Tasks and Functions: Simplifying Hardware Design.
- Meta Description: Use an engaging meta description incorporating the focus keyword.
Example: “Learn how SystemVerilog tasks and functions simplify hardware design with modularization and reusable code blocks. Boost your design efficiency today!” - Header Tags: Use relevant keywords in H2 and H3 tags.
- Internal Linking: Link to other related articles, such as “Introduction to SystemVerilog” or “Best Practices in Hardware Design.”
- External Linking: Cite authoritative sources or documentation for SystemVerilog.
Conclusion
SystemVerilog tasks and functions are indispensable tools for hardware designers. By promoting modularity, enhancing readability, and enabling code reuse, these constructs simplify the complexity of modern hardware design. Following best practices ensures that tasks and functions not only streamline development but also maintain the high-quality standards demanded in hardware engineering.
Embrace the power of tasks and functions to make your SystemVerilog designs efficient and error-free!