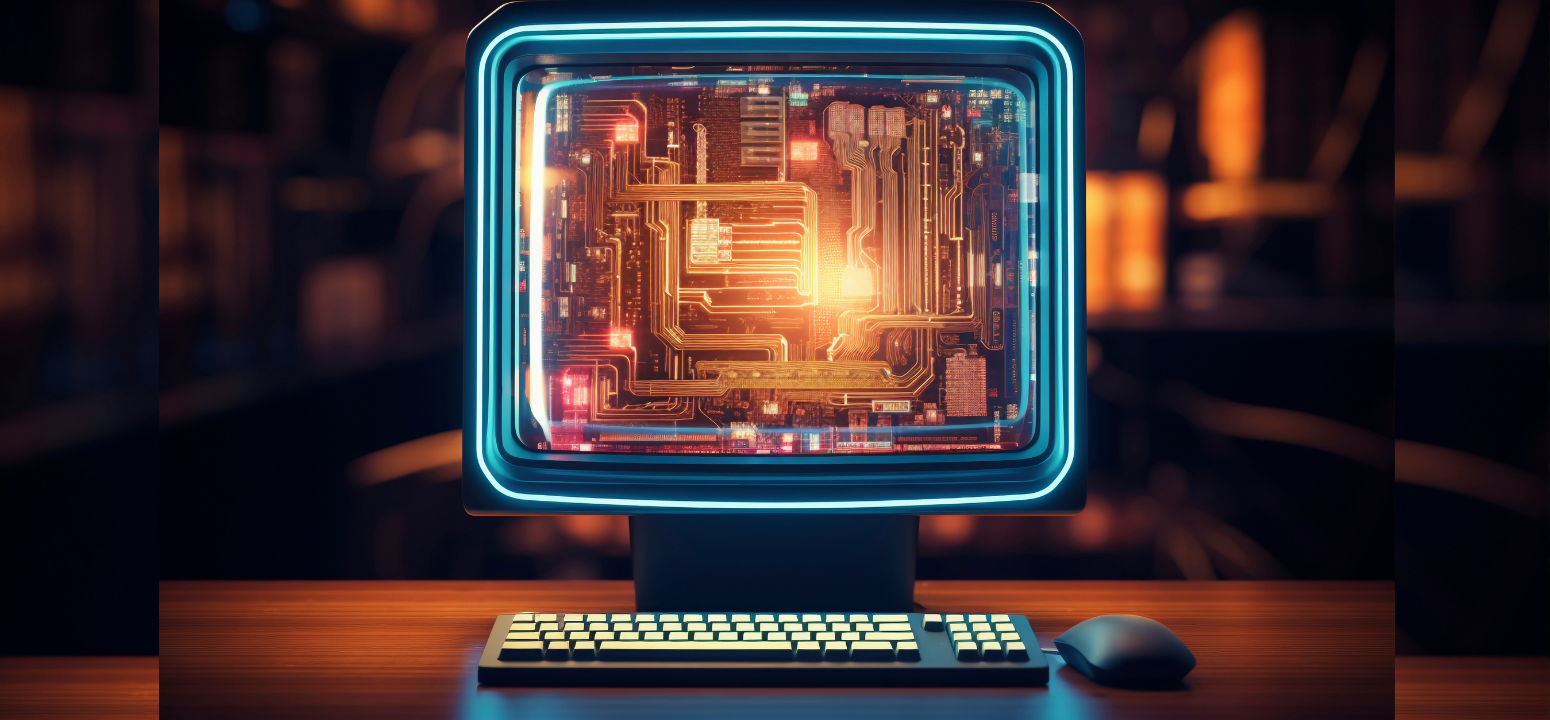
Verilog is one of the most widely used hardware description languages (HDL) in digital design, allowing engineers to model and verify complex systems. To make the most out of Verilog, mastering the creation of reusable modules and setting up testbenches is essential. In this blog post, we’ll cover how to structure Verilog modules for reusability and demonstrate how to set up effective testbenches for verifying designs.
Understanding Verilog Modules
A module in Verilog represents a building block in hardware design. Each module can encapsulate specific functionality, such as an adder, multiplexer, or flip-flop. By creating reusable modules, you can simplify the design process and improve efficiency.
Key Components of a Verilog Module:
- Module Declaration:
This is where the module’s name, inputs, and outputs are defined.
module adder (
input [3:0] a,
input [3:0] b,
output [3:0] sum
);
- Internal Signals:
Inside the module, you can declare additional signals for intermediate logic or for connecting internal components. - Behavioral or Structural Code:
The logic that defines the module’s functionality can be written using either behavioral or structural modeling styles.
- Behavioral Modeling describes the functionality using constructs like
always
blocks. - Structural Modeling describes the design by connecting sub-modules together, simulating real-world connections between hardware components.
- Module Instantiation:
Modules can be instantiated inside other modules to create a hierarchical design, making code reusable across various designs.
adder u_adder (
.a(a),
.b(b),
.sum(sum)
);
Reusable Modules in Verilog
To ensure your Verilog modules are reusable, follow these practices:
- Parameterization:
Use parameters to make modules flexible. This allows for modules to be configured with different widths or functionality without rewriting the code.
module adder #(parameter WIDTH = 4) (
input [WIDTH-1:0] a,
input [WIDTH-1:0] b,
output [WIDTH-1:0] sum
);
- Clear Documentation:
Comment your code thoroughly to ensure that other users can understand and reuse your modules effectively. - Avoid Hardcoding:
Avoid using hardcoded values within the module logic. Use parameters or input signals for values that may change across different designs.
What is a Testbench?
A testbench is a piece of code used to test and verify a Verilog module. It simulates the inputs and observes the outputs of the module under test, ensuring that it behaves as expected. Without proper verification, hardware designs are prone to errors, leading to costly design flaws.
Key Components of a Verilog Testbench
- Module Declaration:
Unlike regular Verilog modules, a testbench has no ports. It directly instantiates the module under test (MUT).
module testbench;
- Input Signal Declarations:
Declare the inputs to the MUT asreg
types since you’ll be driving these signals in the testbench.
reg [3:0] a, b;
wire [3:0] sum;
- Instantiation of the Module Under Test (MUT):
Create an instance of the module that you are verifying.
adder uut (
.a(a),
.b(b),
.sum(sum)
);
- Stimulus Generation:
This is where the test inputs are driven to the module. Typically, stimulus is generated inside aninitial
block that runs at the start of the simulation.
initial begin
a = 4'b0001; b = 4'b0010;
#10;
a = 4'b0100; b = 4'b0011;
#10;
end
- Monitoring Outputs:
Use$monitor
or$display
to print the outputs of the module for observing behavior.
initial begin
$monitor("At time %0t: a = %b, b = %b, sum = %b", $time, a, b, sum);
end
- Simulation Control:
Use$finish
to end the simulation after a certain period or after specific conditions are met.
initial begin
#100;
$finish;
end
Example: Full Verilog Testbench
Let’s put it all together with an example of a testbench for a 4-bit adder:
module testbench;
// Inputs to the module under test (MUT)
reg [3:0] a, b;
wire [3:0] sum;
// Instantiate the adder module
adder uut (
.a(a),
.b(b),
.sum(sum)
);
// Stimulus generation
initial begin
a = 4'b0001; b = 4'b0010;
#10;
a = 4'b0100; b = 4'b0011;
#10;
a = 4'b1111; b = 4'b0001;
#10;
$finish;
end
// Monitor output
initial begin
$monitor("At time %0t: a = %b, b = %b, sum = %b", $time, a, b, sum);
end
endmodule
Importance of Testbenches in Design Verification
Testbenches are crucial in catching design errors early. They provide a virtual environment to verify the logic and timing of a design, reducing the risk of costly hardware bugs. By automating the testing process, you can save time and increase design reliability.
Conclusion
Creating reusable Verilog modules and setting up robust testbenches are essential skills for any digital design engineer. By structuring your code for reusability, you can simplify future design tasks, while testbenches ensure your designs function as expected before they reach the implementation stage. Mastering these techniques will help you become more efficient and effective in your Verilog-based projects.
Also Read : fpga architecture in vlsi
To know more about VLSI Course , SuccessBridge VLSI training institute. You can begin your VLSI career by enrolling in the placement-assisted live courses available at SuccessBridge We offer various VLSI online courses. We offer VLSI Physical Design course, Design Verification course, DFT Training , Chip design course many more. Explore VLSI Courses From The Leaders In VLSI Training
.